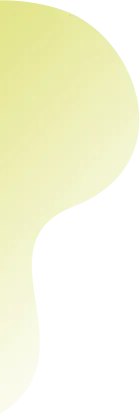
Spring is coming! – ArangoDB meets Spring Data
This year we got a lot of requests from our customers to provide Spring Data support for ArangoDB. So we listened and teamed up with one of our bigger customers from the financial sector to develop a Spring Data implementation for ArangoDB. We have also made an extensive demo on how to use Spring Data ArangoDB with an example data set of Game of Thrones characters and locations. So, Spring is not only coming… it is already there!
What is the Spring Framework?
The Spring Framework is an open source Java application framework which provides an Inversion of Control (IoC) Container to manage Plain-Old-Java-Objects (POJO) through Dependency Injection (DI). The Spring Framework includes a wide range of modules providing several services. One interesting module for us is Spring Data which provides a Spring like programming model for data access. There are already a lot of subprojects of Spring Data which specify data access to different specific database technologies and now ArangoDB joins in.
Why did it take some time?
To successfully implement our Spring Data module for ArangoDB we needed a solid base with our team and with our Java driver which runs under the hood. In the last two years, we expanded our team of developers who are well versed in the Java world. With their help we implemented a completely new Java driver with a more intuitive and object oriented API, but also several new features like VelocyStream support, multi-document operations, automatic fallback and, with the current release, built-in load balancing. Especially the load balancing and fallback features were a high priority for our customers running ArangoDB in a cluster setup. With this preliminary work, we started implementing our Spring Data module this year in close teamwork with two great developers from our customer. (Thanks for your great work, guys!)
Features
Spring Data ArangoDB provides a solution for all core concepts of Spring Data. With ArangoTemplate you are able to perform common database operations from managing databases, collections and graphs to single or batch CRUD operations. This includes annotation based object mapping of POJOs to VelocyPack documents (ArangoDBs internal storage format) and exception translation into data access exceptions used in Spring. You are also able to write repository interfaces which will be automatically implemented. Within these repository interfaces, you can implement custom methods from which AQL queries will be derived. With this feature, you can perform a wide range of queries with filter conditions, joins, graph traversals and even geospatial queries. It also supports passing AQL bind parameters as parameters in your method. But you can still write your AQL queries on your own and attach it with the @Query annotation to your custom method.
Example
Configuration
Configure the connection to ArangoDB server and enable Spring Data ArangoDB repositories.
@Configuration
@EnableArangoRepositories(basePackages = { "com.arangodb.spring.demo" })
public class DemoConfiguration extends AbstractArangoConfiguration {
@Override
public Builder arango() {
return new ArangoDB.Builder().host("localhost", 8529).user("root").password(null);
}
@Override
public String database() {
return "spring-demo";
}
}
Entities
Create and annotate your objects.
@Document
public class Character {
@Id
private String id;
private Integer age;
@Relations(edges = ChildOf.class, lazy = true)
private Collection<Character> childs;
}
@Edge
public class ChildOf {
@Id
private String id;
@From
private Character child;
@To
private Character parent;
}
Repositories
Create repository interfaces and implement custom methods.
public interface CharacterRepository extends ArangoRepository<Character> {
Iterable<Character> findByChildsAgeBetween(int lowerBound, int upperBound);
}
public interface ChildOfRepository extends ArangoRepository<ChildOf> {
}
Usage
@Autowired CharacterRepository characterRepo;
@Autowired ChildOfRepository childOfRepo;
List<Character> characters = ...
characterRepo.save(characters)
List<ChildOf> edges = ...
childOfRepo.save(edges);
Iterable<Character> childsBetween16a20 = repo.findByChildsAgeBetween(16, 20);
3 Comments
Leave a Comment
Get the latest tutorials, blog posts and news:
Hi Mark, is ArangoDB actively maintaining the Spring Data access for ArangoDB or is this your work out of passion?
Hi Bo, yes SpringData is actively maintained and can be found here: https://github.com/arangodb/spring-data
Hi, do we have spring batch support for importing bulk data