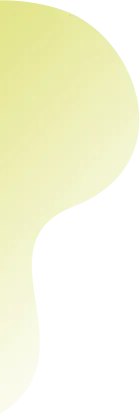
ArangoDB vs. Neo4J
Estimated reading time: 7 minutes
Update: https://arangodb.com/2023/10/evolving-arangodbs-licensing-model-for-a-sustainable-
future/
Last October the first iteration of this blog post explained an update to ArangoDB’s 10-year-old license model. Thank you for providing feedback and suggestions. As mentioned, we will always remain committed to our community and hence today, we are happy to announce yet another update that integrates your feedback.
Your ArangoDB Team
ArangoDB as a company is firmly grounded in Open Source. The first commit was made in October 2011, and today we're very proud of having over 13,000 stargazers on GitHub. The ArangoDB community should be able to enjoy all of the benefits of using ArangoDB, and we have always offered a completely free community edition in addition to our paid enterprise offering.
With the evolving landscape of database technologies and the imperative to ensure ArangoDB remains sustainable, innovative, and competitive, we’re introducing some changes to our licensing model. These alterations will help us continue our commitment to the community, fuel further cutting-edge innovations and development, and assist businesses in obtaining the best from our platform. These alterations are based on changes in the broader database market.
Upcoming Changes
The changes to the licensing are in two primary areas:
- Distribution and Managed Services
- Commercial Use of Community Edition
Distribution and Managed Services
Effective version 3.12 of ArangoDB, the source code will replace its existing Apache 2.0 license with the BSL 1.1 for 3.12 and future versions.
BSL 1.1 is a source-available license that has three core tenets, some of which are customizable and specified by each licensor:
- BSL v.1.1 will always allow copying, modification, redistribution, non-commercial use, and commercial use in a non-production context.
- By default, BSL does not allow for production use unless the licensor provides a limited right as an “Additional Use Grant”; this piece is customizable and explained below.
- BSL provides a Change Date usually between one to four years in which the BSL license converts to a Change License that is open source, which can be GNU General Public License (GPL), GNU Affero General Public License (AGPL), or Apache, etc.
ArangoDB has defined our Additional Use Grant to allow BSL-licensed ArangoDB source code to be deployed for any purpose (e.g. production) as long as you are not (i) creating a commercial derivative work or (ii) offering or including it in a commercial product, application, or service (e.g. commercial DBaaS, SaaS, Embedded or Packaged Distribution/OEM). We have set the Change Date to four (4) years, and the Change License to Apache 2.0.
These changes will not impact the majority of those currently using the ArangoDB source code but will protect ArangoDB against larger companies from providing a competing service using our source code or monetizing ArangoDB by embedding/distributing the ArangoDB software.
As an example, If you use the ArangoDB source code and create derivative works of software based on ArangoDB and build/package the binaries yourself, you are free to use the software for commercial purposes as long as it is not a SaaS, DBaaS, or OEM distribution. You cannot use the Community Edition prepackaged binaries for any of the purposes mentioned above.
Commercial Use of Community Edition
We are also making changes to our Community Edition with the prepackaged ArangoDB binaries available for free on our website. Where before this edition was governed by the same Apache 2.0 license as the source code, it will now be governed by a new ArangoDB Community License, which limits the use of community edition for commercial purposes to a 100GB limit on dataset size in production within a single cluster and a maximum of three clusters.
Commercial use describes any activity in which you use a product or service for financial gain. This includes whenever you use software to support your customers or products, since that software is used for business purposes with the intent of increasing sales or supporting customers. This explicitly does not apply to non-profit organizations.
As an example, if you deploy software in production that uses ArangoDB as a database, the database size is under 100 GB per cluster, and it is limited to a maximum of three clusters within an organization. Even though the software is commercially used, you have no commercial obligation to ArangoDB because it falls under the allowed limits. Similarly, non-production deployments such as QA, Test, and Dev using community edition create no commercial obligations to ArangoDB.
Our Enterprise Edition will continue to be governed by the existing ArangoDB Enterprise License.
What should Community users do?
The license changes will roll out and be effective with the release of 3.12 slated for the end of Q1 2024, and there will be no immediate impact to any releases prior to 3.12. Once the license changes are fully applied, there will be a few impacts:
- If you are using Community Edition or Source Code for your managed service (DBaaS, SaaS), you will be unable to do so for future versions of ArangoDB starting with version 3.12.
- If you are using Community Edition or Source Code and distributing it to your customers along with your software, you will be unable to do so for future versions of ArangoDB starting with version 3.12.
- If you are using the Community Edition for commercial purposes for any production deployment either storing greater than 100 GB of data per cluster or having more than three clusters or both - you are required to have a commercial agreement with ArangoDB starting with version 3.12.
If any of these apply to you and you want to avoid future disruption, we encourage you to contact us so that we can work with you to find a commercially acceptable solution for your business.
How is ArangoDB easing the transition for community users with this change?
ArangoDB is willing to make concessions for community users to help them with the transition and the license change. Our joint shared goal is to both enable ArangoDB to continue commercially as the primary developer of the CE edition and still allow our CE users to have successful deployments that meet their business and commercial goals. Support from Arango and help with ongoing help with your deployments (Our Customer Success Team) allows us to maintain the quality of deployments and, ultimately, a more satisfying experience for users.
We do not intend to create hardship for the community users and are willing to discuss reasonable terms and conditions for commercial use.
ArangoDB can offer two solutions to meet your commercial use needs:
- Enterprise License: Provide a full-fledged enterprise license for your commercial use with all the enterprise features along with Enterprise SLA and Support.
- Community Transition We do not intend to create hardship for the community users and hence created a 'CE Transition Fund', which can be allocated by mutual discussion to ease the transition. This will allow us to balance the value that CE brings to an organization and the Support/Features available.
Summary
Our commitment to open-source ideals remains unshaken. Adjusting our model is essential to ensure ArangoDB’s longevity and to provide you with the cutting-edge features you expect from us. We continue to uphold our vision of an inclusive, collaborative, and innovative community. This change ensures we can keep investing in our products and you, our valued community.
Frequently Asked Questions
1. Does this affect the commercially packaged editions of your software such as Arango Enterprise Edition, and ArangoGraph Insights Platform?
No, this only affects ArangoDB source code and ArangoDB Community Edition.
2. Whom does this change primarily impact?
This has no effect on most paying customers, as they already license ArangoDB under a commercial license. This change also has no effect on users who use ArangoDB for non-commercial purposes. This change affects open-source users who are using ArangoDB for commercial purposes and/or distributing and monetizing ArangoDB with their software.
3: Why change now?
ArangoDB 3.12 is a breakthrough release that includes improved performance, resilience, and memory management. These highly appealing design changes may motivate third parties to fork ArangoDB source code in order to create their own commercial derivative works without giving back to the developer community. We feel it is in the best interest of the community and our customers to avoid that outcome.
4: In four years, after the Change Date, can I make my own commercial product from ArangoDB 3.12 source code under Apache 2.0?
Yes, if you desire.
5: Is ArangoDB still an Open Source company?
Yes. While the BSL 1.1 is not an official open source license approved by the Open Source Initiative (OSI), we still license a large amount of source code under an open source license such as our Drivers, Kube-Arango Operator, Tools/Utilities, and we continue to host ArangoDB-related open source projects. Furthermore, the BSL only restricts the use of our source code if you are trying to commercialize it. Finally, after four years, the source code automatically converts to an OSI-approved license (Apache 2.0).
6: How does the license change impact other products, specifically the kube-arango operator?
There are two versions of the kube-arango operator: the Community and the Enterprise versions. At this time there are no plans to change licensing terms for the operator. The operator will, however, automatically enforce the licensing depending upon the ArangoDB version under management (enterprise or community).
Get the latest tutorials, blog posts and news: